💡 I included the link to the example repository at the conclusion of this tutorial.
For some webs, authentication is one of the most important thing. And maybe sometimes our websites also need to easily gain more engagement by eliminating a bit hassle by letting users login to the web easily using their other platform account.
Google is one of the most widely used platform by people around the globe. This can be used as our advantage if we can provide a one-click login feature by using Google account, without making users to fill data manually that can be take about 5 minutes before they can use our website.
There is a technology called OAuth (Open Authorization), it is an open standard for access delegation. With OAuth we can login to websites or applications using other website or application account without giving or creating new passwords. This can make granting access to our website easier and more secure for user rather than to create an account on our website.
Laravel provides a package to authenticate with OAuth providers using Laravel Socialite. Currently Socialite supports authentication via some well-known platform like Facebook, Twitter, LinkedIn, Google, GitHub, GitLab, and Bitbucket.
This time I will share about how we can make Google OAuth login using Laravel Socialite.
Installation
Laravel Installation
First you want to install a Laravel app first.
composer create-project laravel/laravel blog-google-oauth-laravel-socialite
Laravel Socialite Installation
Get started with Socialite by adding the package using Composer package manager.
composer require laravel/socialite
Configuration
Typically we need credentials for OAuth providers we use. For Google, we need to have GOOGLE_CLIENT_ID
and GOOGLE_CLIENT_SECRET
for our web. We can get those credentials by creating Google API Credentials in Google APIs Console.
Create Google API Credentials
Step 1: Open the Credentials Page
By visiting Google APIs console. This is how the page looks when this article is written.
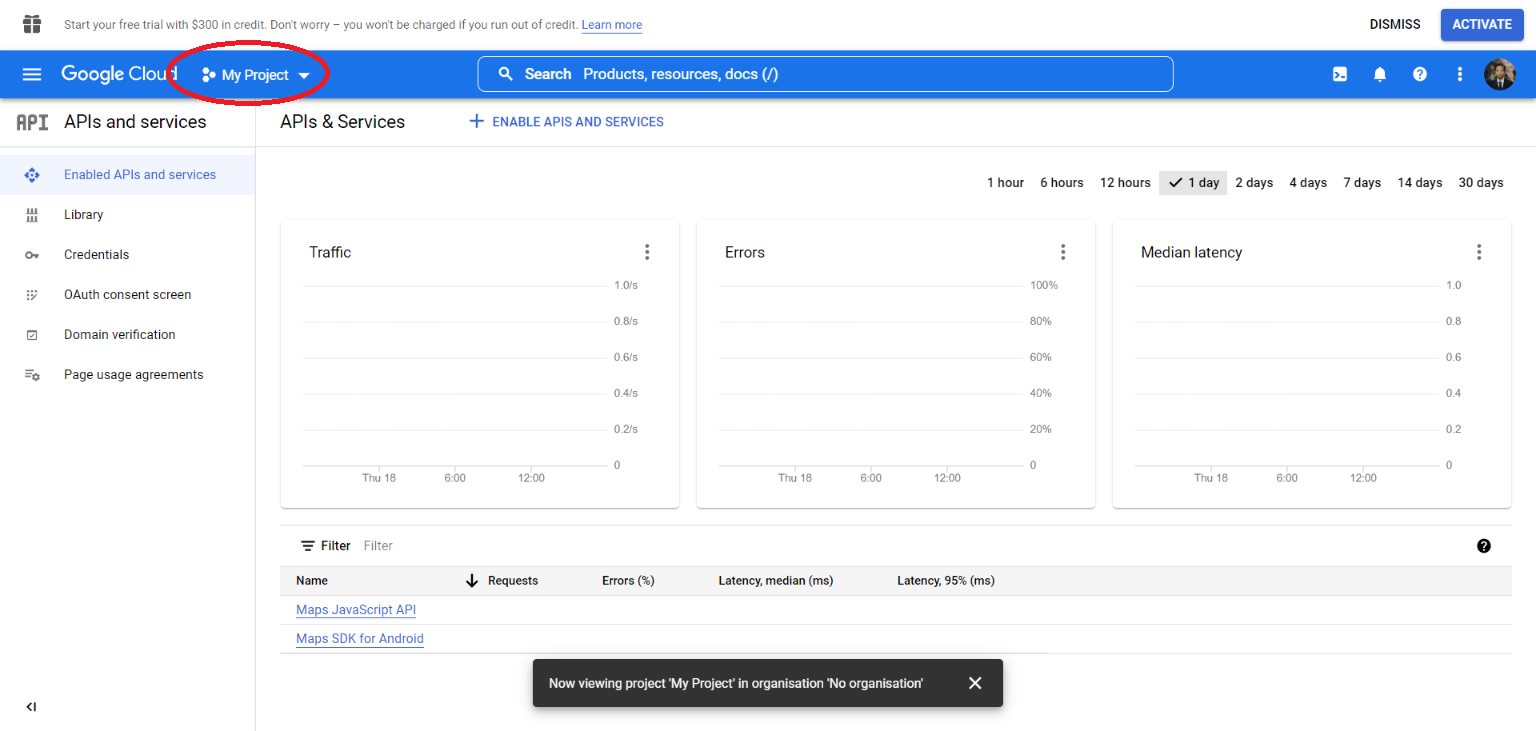
Step 2: Create a Project
Click the dropdown that circled above, and then click “New Project”
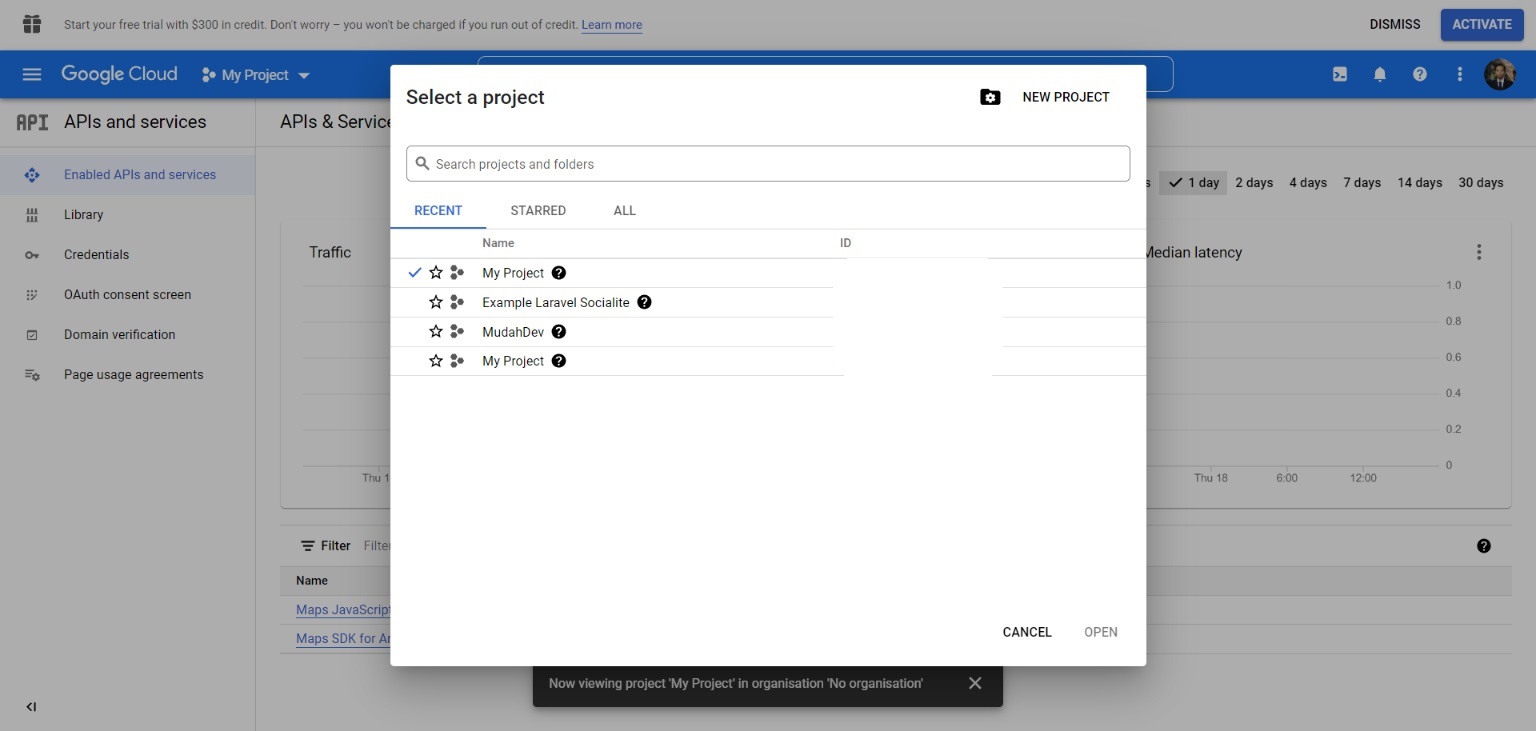
Fill the detail about your project, here is the example and then click “Create”.
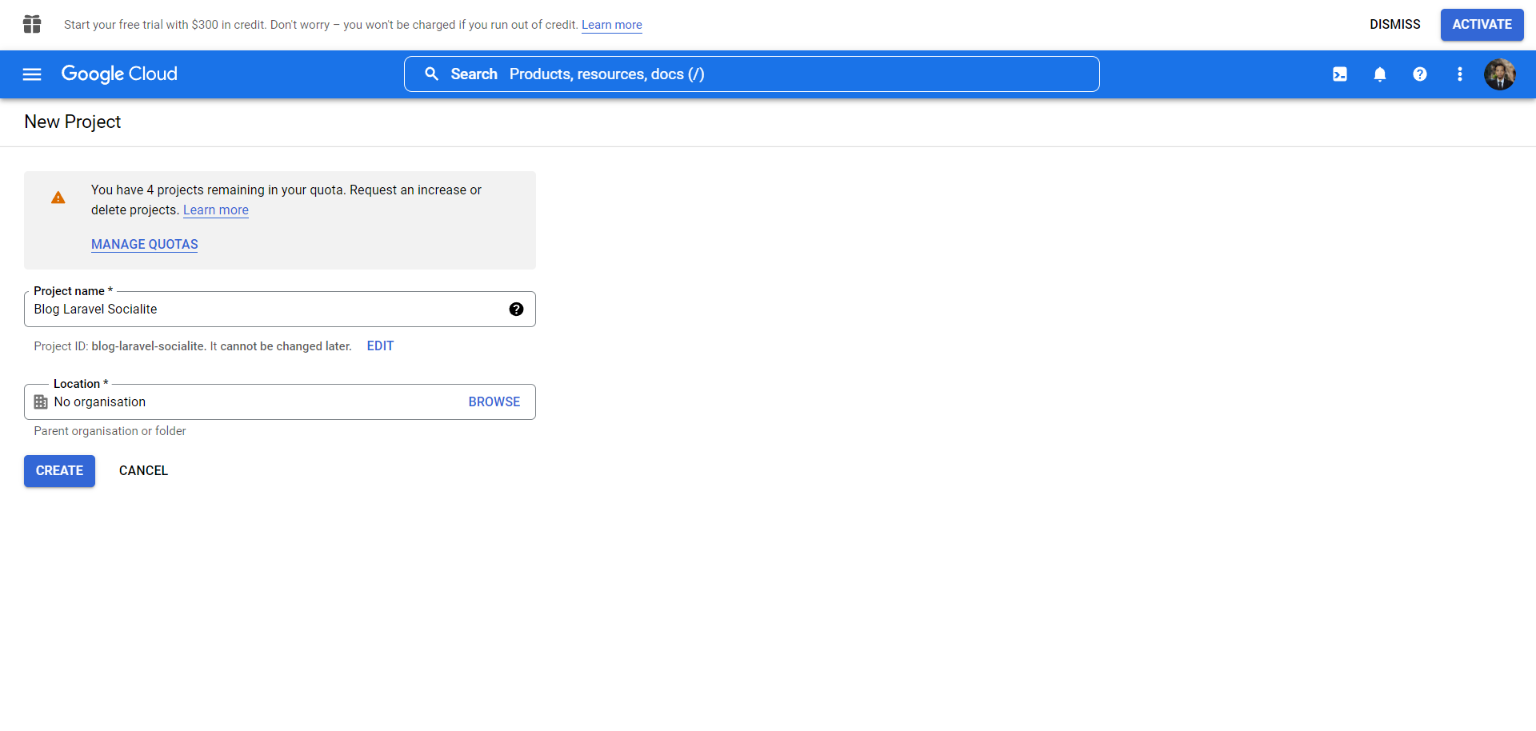
Wait until project successfully created
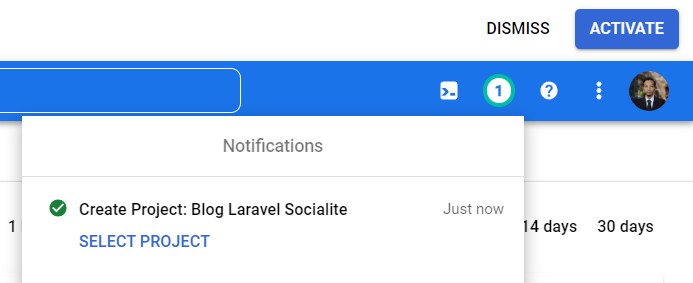
Step 3: Switch to the Project
Switch to our newly project by clicking the droprown of project that is displayed in the navbar above, click the project and click “Open” button.
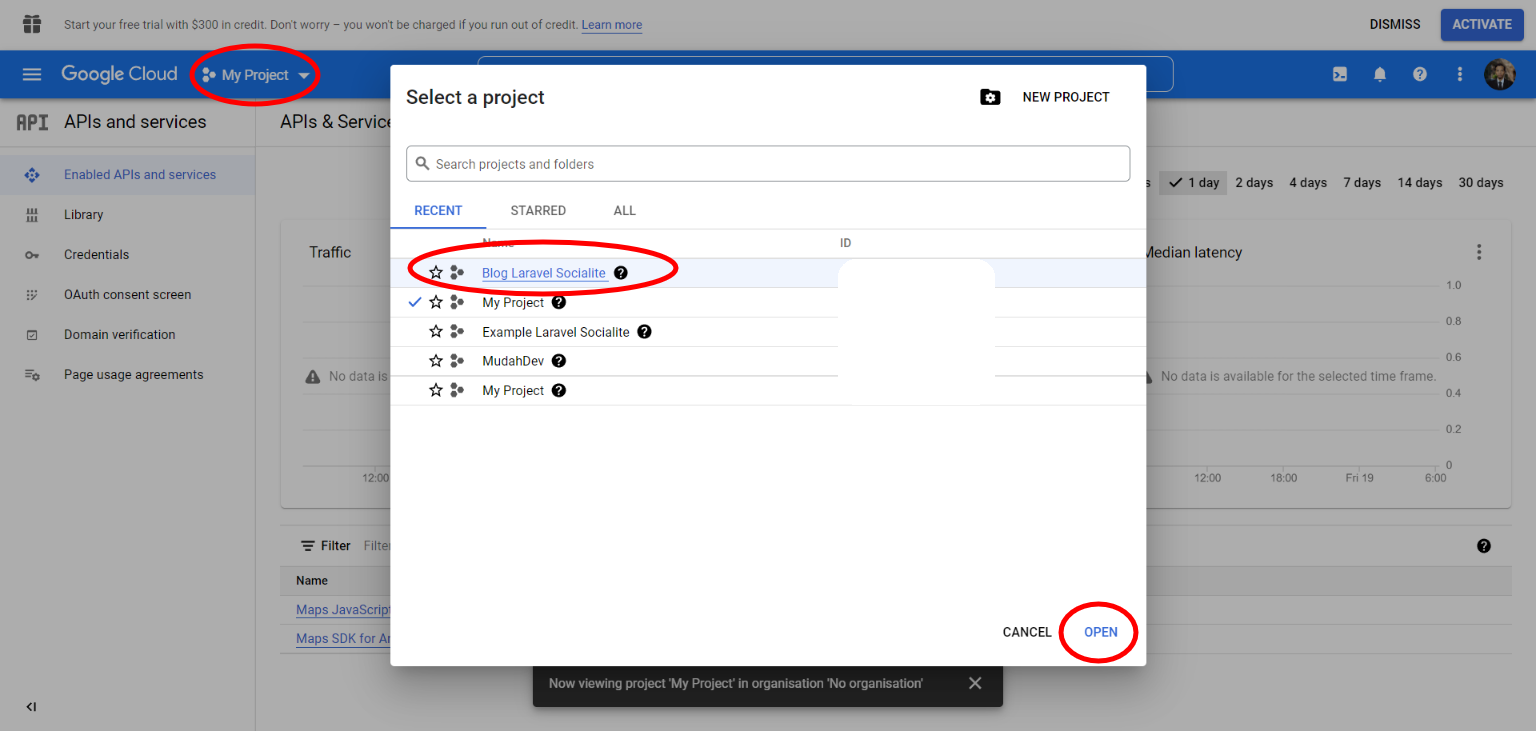
Step 4: Try to Create OAuth Client ID Credential
Create the credential by choosing “Credentials” in the side bar, click “Create Credentials” button and choose “OAuth Client ID”.
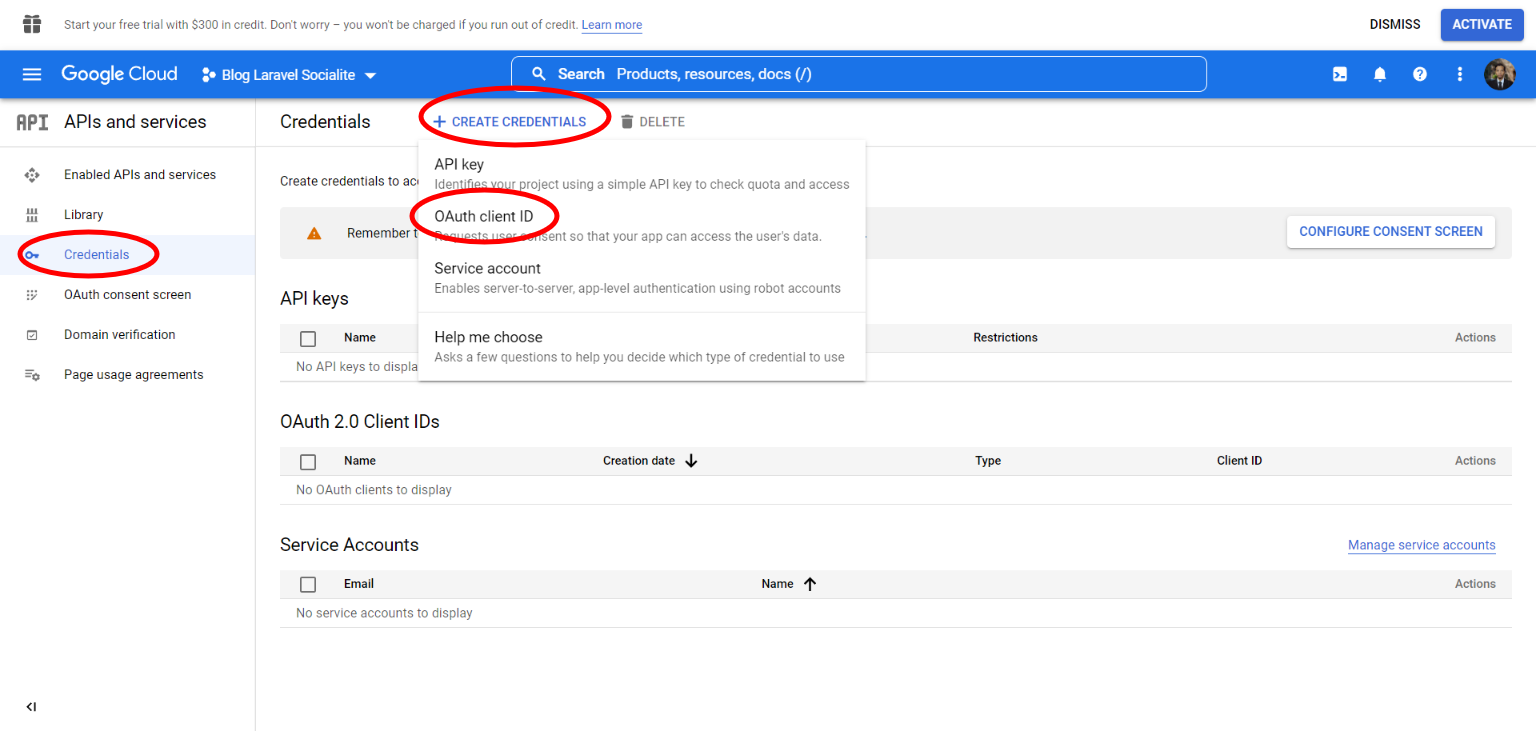
Step 5: Configure Consent Screen
If it says we need to configure consent screen first then click the button
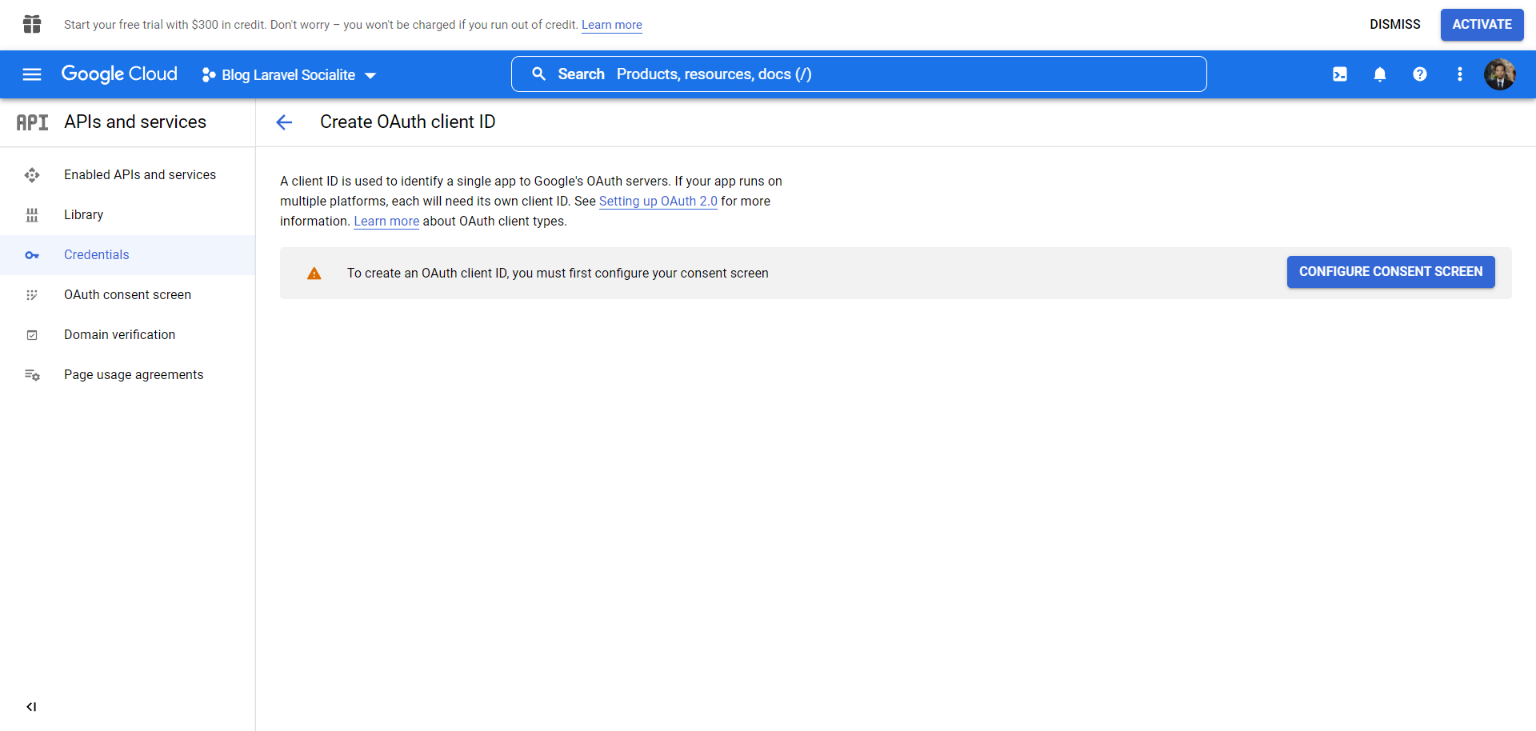
We will be redirected to the screen and choose based on your needs, here I will choose “External” option then create.
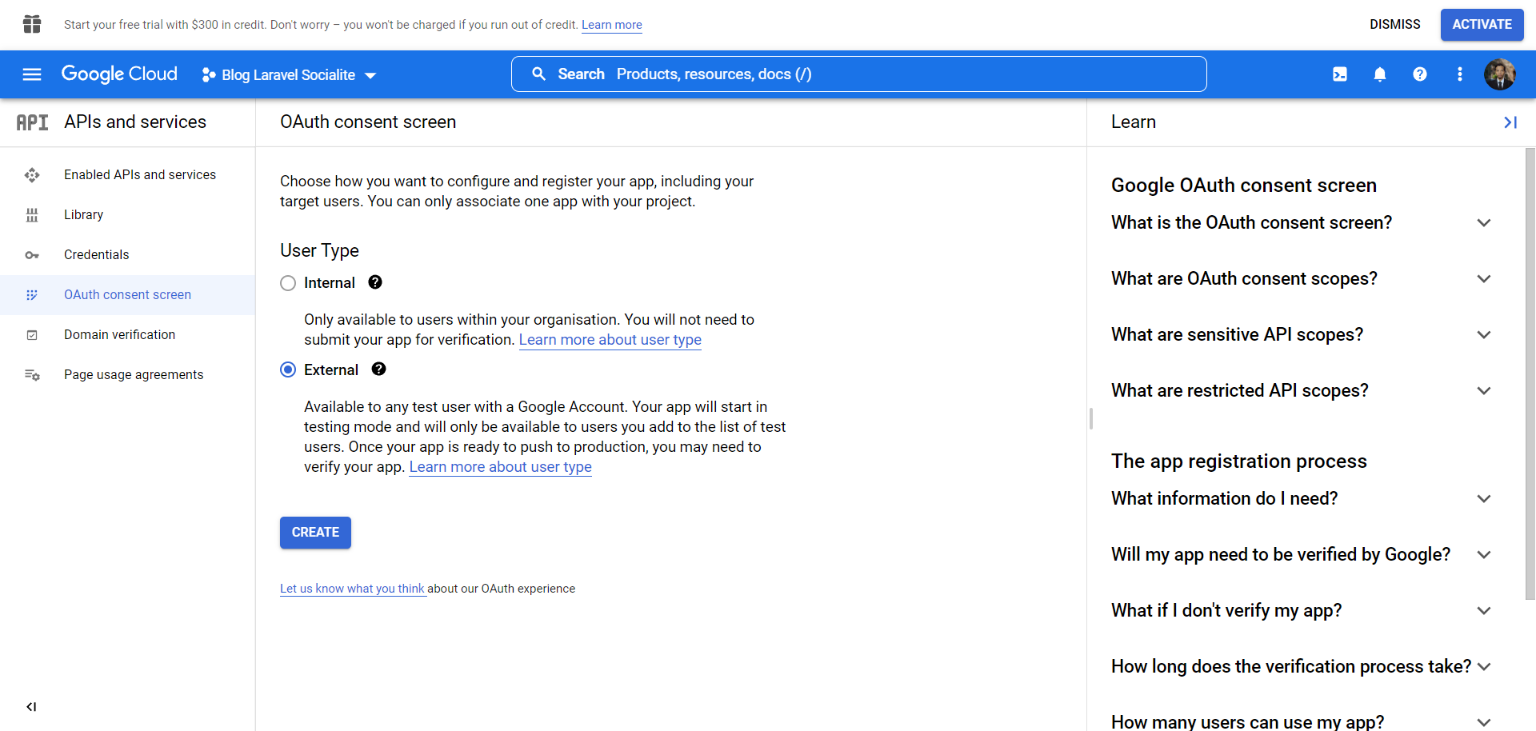
Step 6: Edit App Registration
Fill the form with. Here I just fill the App Name, user support email, and developer contact information, and then click “Save and Continue”.
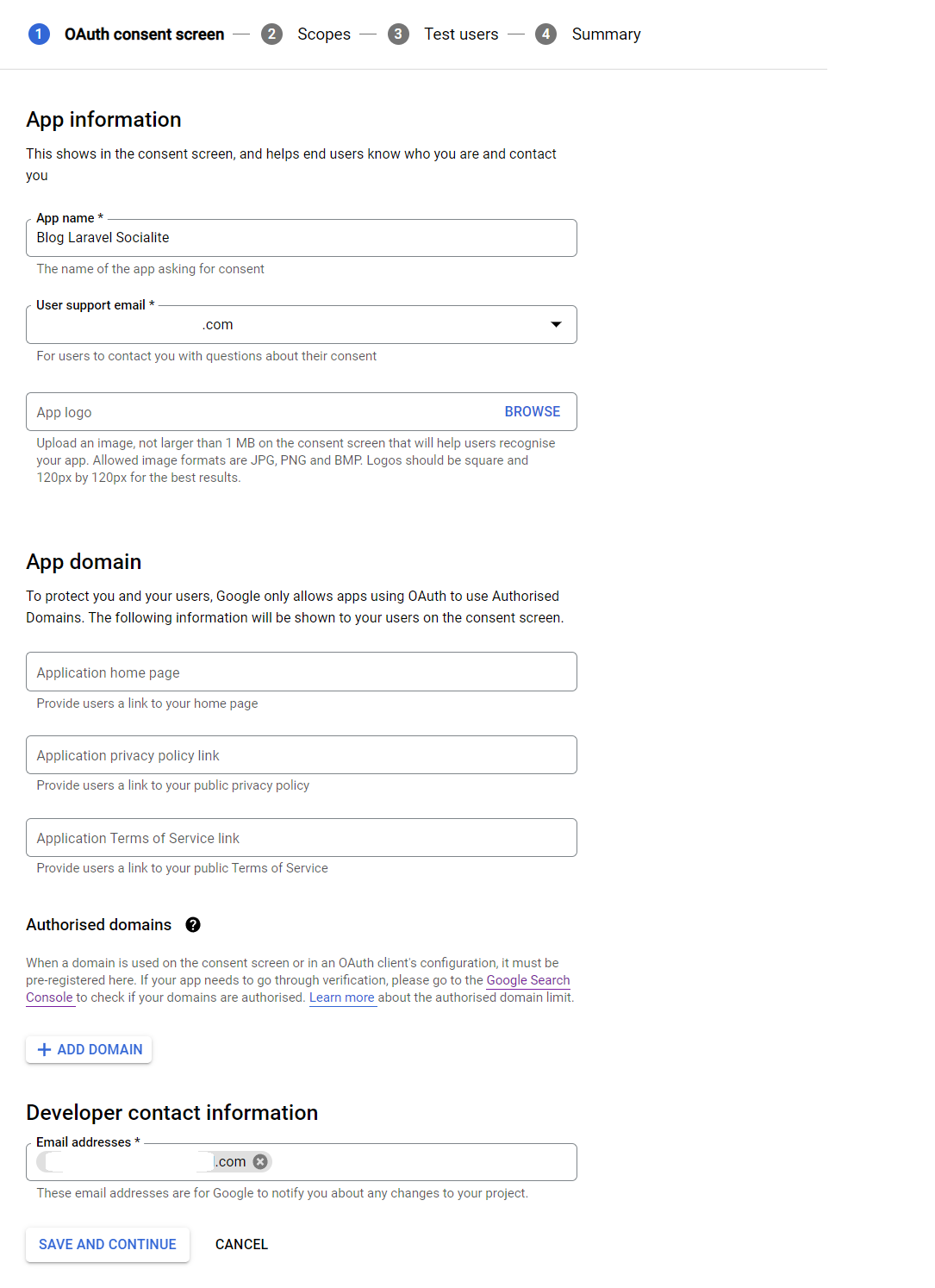
You can continue to fill the form but in our simple app it’s not necessary. I proceed to Credentials page and try to “Create OAuth Client ID” once again.
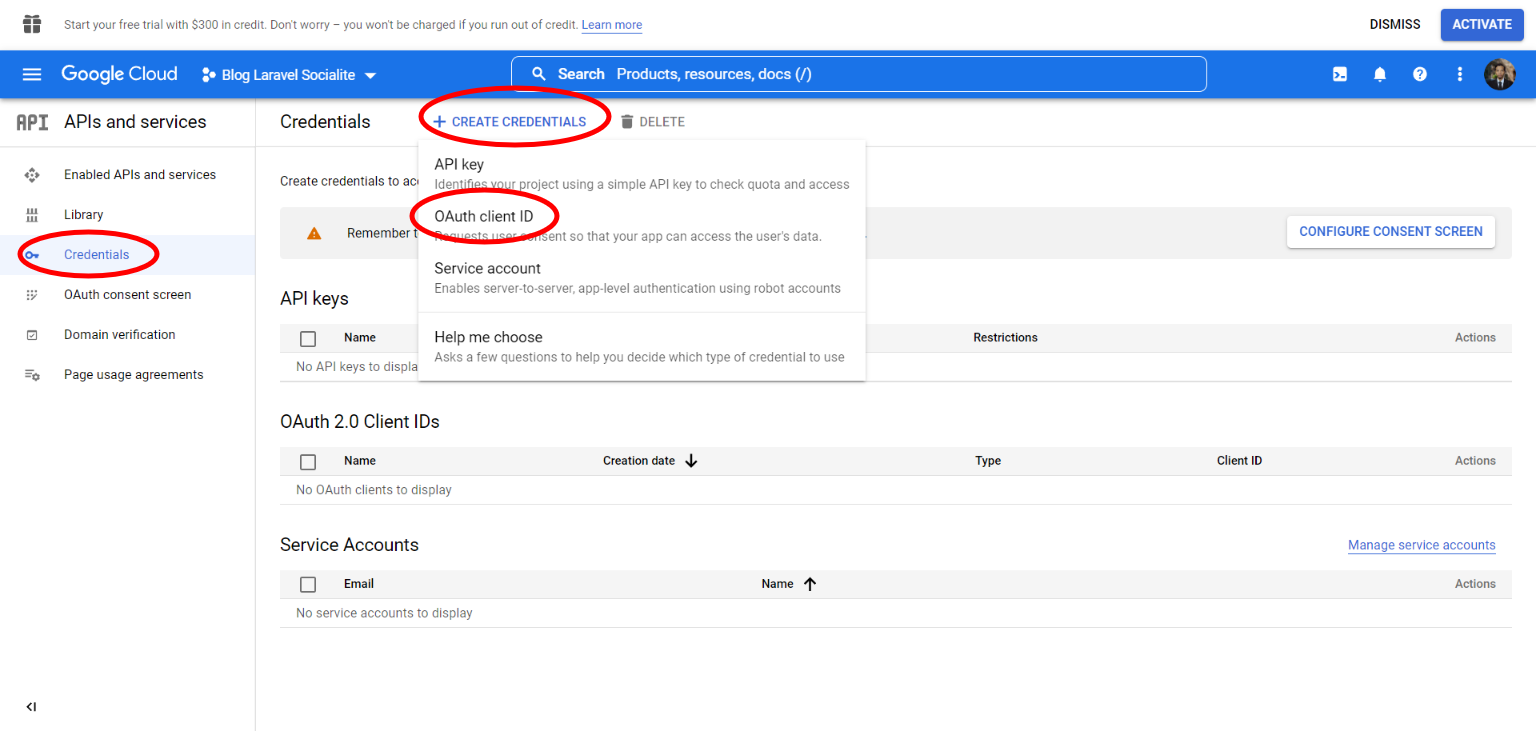
Step 7: Create OAuth Client ID Credential
Here we can fill the detail about our app and fill the authorised redirect URIs. This is the URI that we will use to redirect user after they choose their Google account to login to our web. For example here I use http://127.0.0.1:8000/callback/google
for the callback URI.
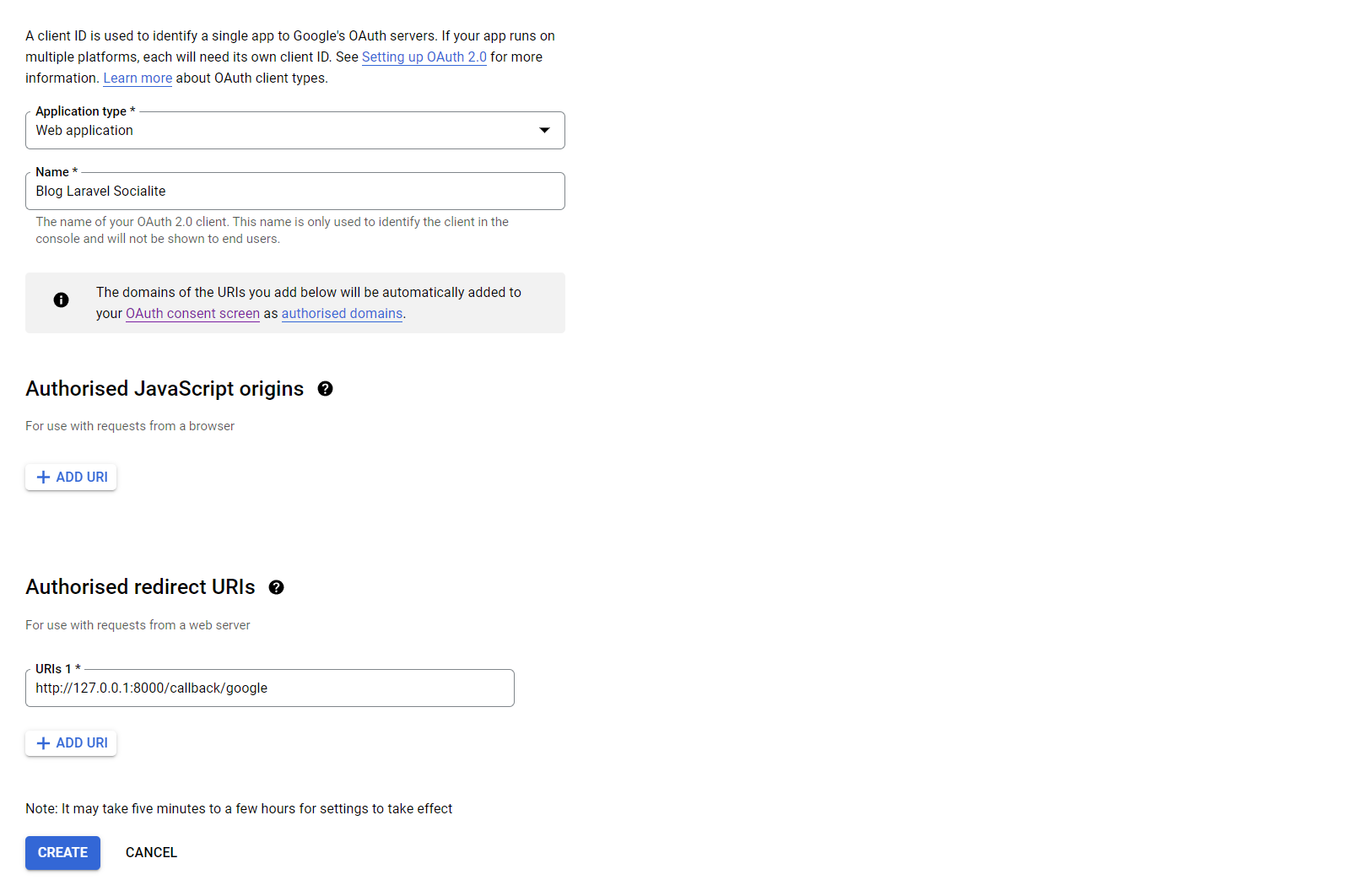
Step 8: OAuth Client Created
Now we get the Client ID and the Client Secret.
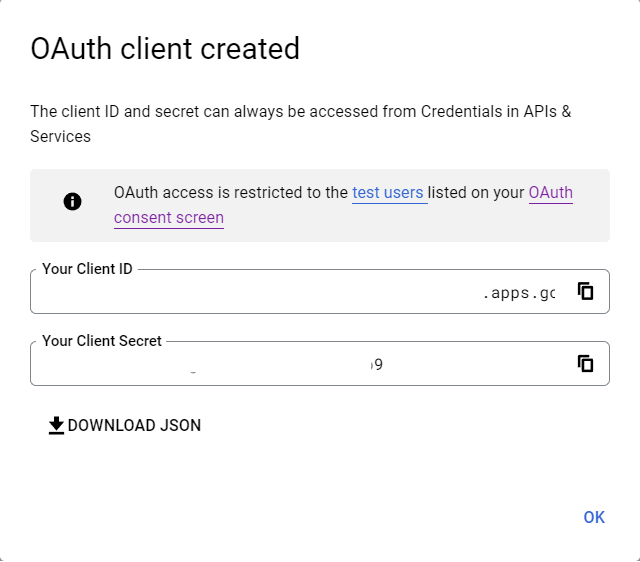
Configuration with Laravel
Step 1: Place the Credentials in .env
Place the Client ID and Client Secret key we got just now in GOOGLE_CLIENT_ID
key and GOOGLE_CLIENT_SECRET
key in the Laravel .env
respectively. Another key to add in .env
is GOOGLE_REDIRECT
, fill it with our callback URI we use in the Google API Console.
GOOGLE_CLIENT_ID=blabla.apps.googleusercontent.com
GOOGLE_CLIENT_SECRET=BLA-BLA-BLA
GOOGLE_REDIRECT=http://127.0.0.1:8000/callback/google
Step 2: Place the Google .env key in config/services.php
The credentials should be placed in our config/services.php
configuration file of Laravel.
// config/services.php
return [
//...
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT')
],
];
Read also: Laravel Api Documentation Generator With OpenAPI/Swagger Using DarkaOnLine/L5-Swagger
Database Configuration
Step 1: Create the Migration
Create two columns named social_id
and social_type
in users
table. We can add to the users
table migration or add a migration for adding both columns
php artisan make:migration "add social id and social type field to users table"
Add these codes to the new migration file
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function (Blueprint $table) { // add the below column to "users" table
$table->string('social_id')->nullable(); // add social_id column with varchar type
$table->string('social_type')->nullable(); // add social_type column with varchar type
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('social_id');
$table->dropColumn('social_type');
});
}
};
Step 2: Add social_id and social_type to $fillable in User Model
Add these values to $fillable
.
// app\Models\User.php
protected $fillable = [
'name',
'email',
'password',
'social_id',
'social_type'
];
Step 3: Migrate the Database Migration
Create the blog_google_auth_laravel_socialite
database or your preferred database name for this Laravel app.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=blog_google_auth_laravel_socialite
DB_USERNAME=root
DB_PASSWORD=
Then run the database migration command.
php artisan migrate
Integration with Authentication Feature
We will use Laravel Breeze as an example of our existing authentication implementation.
Install Laravel Breeze
Step 1: Install Laravel Breeze Package
Install it with the following command:
composer require laravel/breeze --dev
Step 2: Install the Laravel Breeze Blade Stack
Here I will use the Blade stack for Laravel Breeze so I will run the following command:
php artisan breeze:install
Step 3: Run the NPM Command
We need to run the npm install
and then npm run dev
command.
npm install
npm run dev
Step 4: Run Our Laravel Web
Run the local development server in another terminal
php artisan serve
Our web can be visited in http://127.0.0.1:8000
Step 5: Check the Authentication Feature
Check the home, login, and dashboard page.
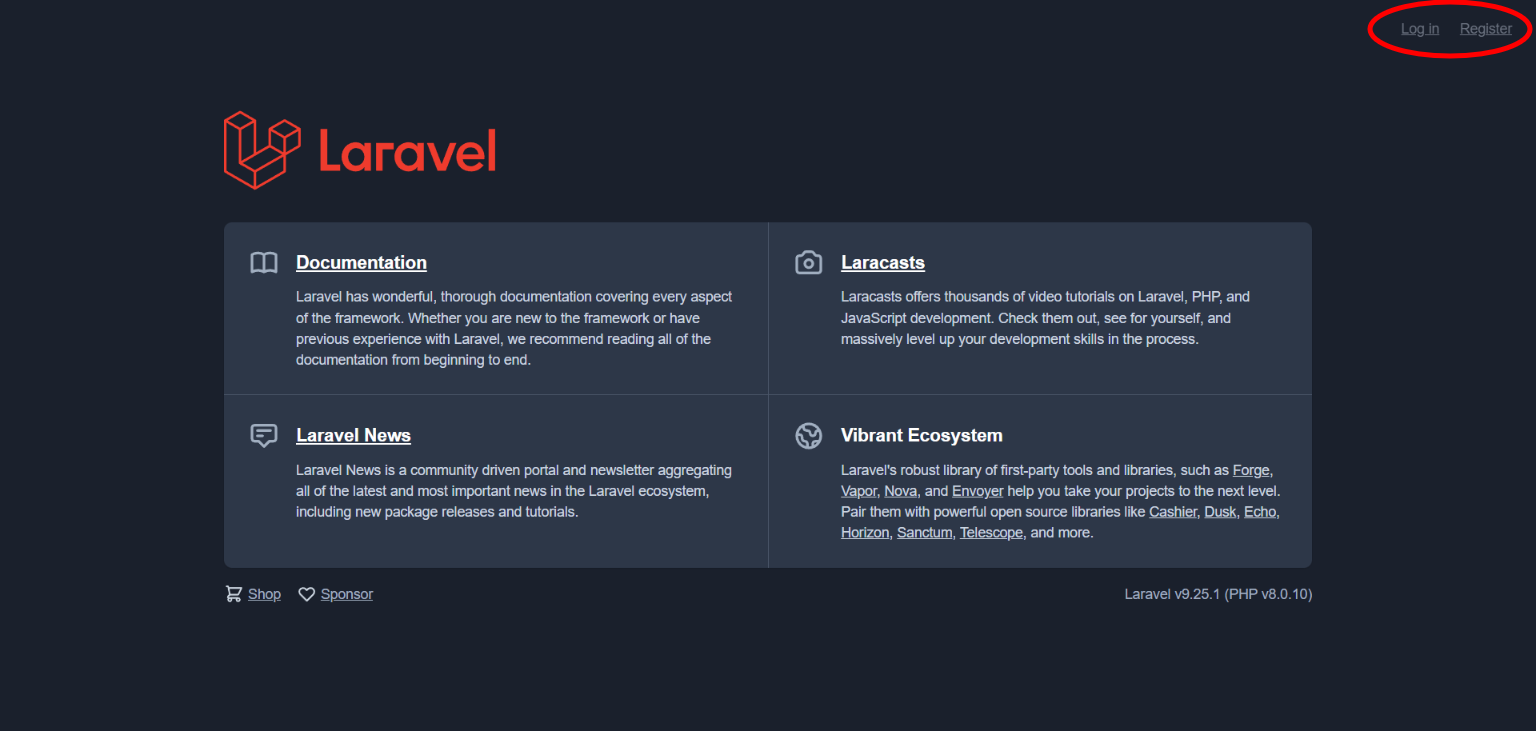
To login to dashboard we need to create an account first, we can create it in register page.
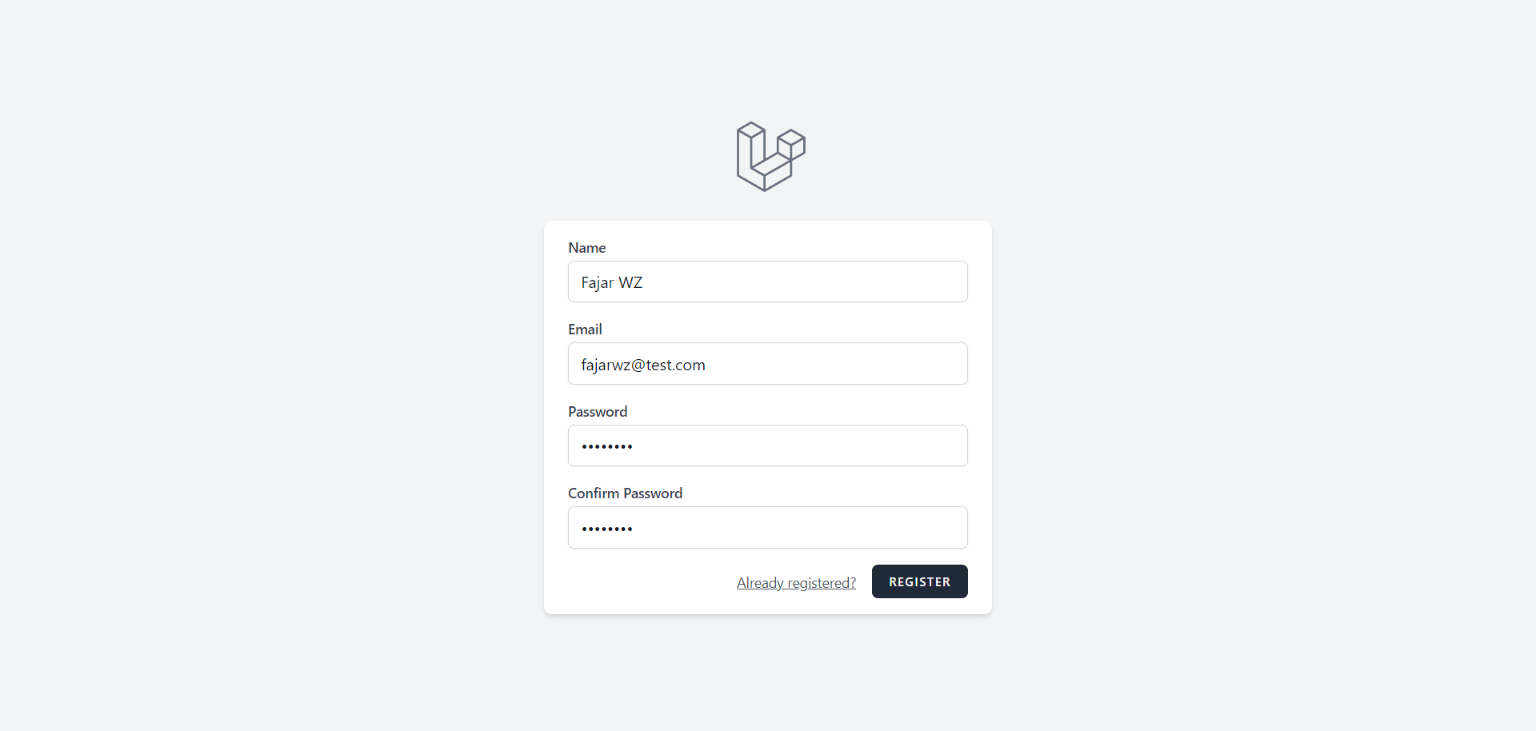
This is how the Dashboard page looks
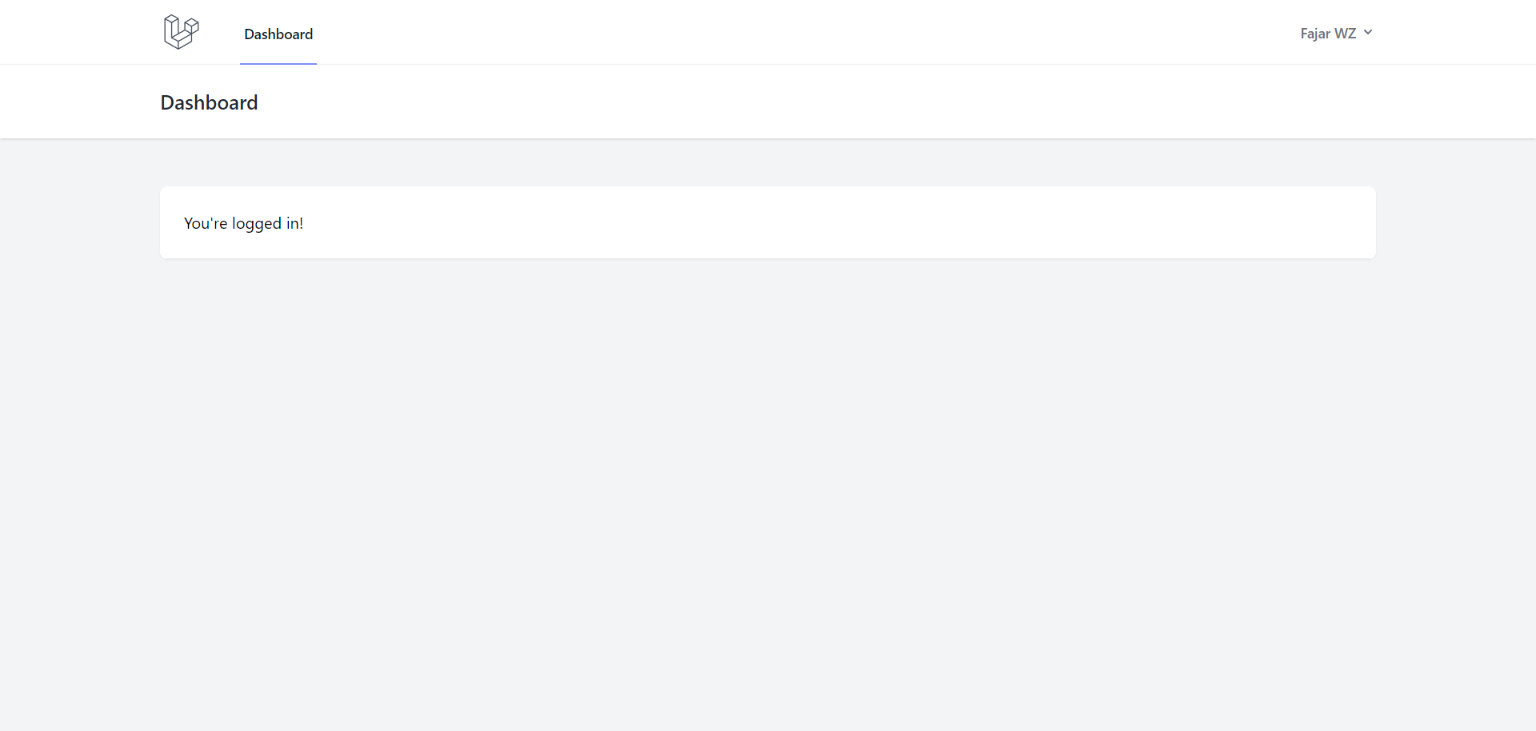
Click the name with dropdown in the navbar and click “Logout” to logout from the web.
Create the Login With Google Button and Implement it
We will start creating the “login with Google” feature.
Step 1: Create the “Redirect to Google” and callback Function in Our Controller
First create the function in another controller. Here I create the controller named GoogleSocialiteController.php
.
php artisan make:controller Auth/GoogleSocialiteController
Create the function below:
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Models\User;
use Auth;
use Exception;
use Socialite;
class GoogleSocialiteController extends Controller
{
public function redirectToGoogle()
{
// redirect user to "login with Google account" page
return Socialite::driver('google')->redirect();
}
public function handleCallback()
{
try {
// get user data from Google
$user = Socialite::driver('google')->user();
// find user in the database where the social id is the same with the id provided by Google
$finduser = User::where('social_id', $user->id)->first();
if ($finduser) // if user found then do this
{
// Log the user in
Auth::login($finduser);
// redirect user to dashboard page
return redirect('/dashboard');
}
else
{
// if user not found then this is the first time he/she try to login with Google account
// create user data with their Google account data
$newUser = User::create([
'name' => $user->name,
'email' => $user->email,
'social_id' => $user->id,
'social_type' => 'google', // the social login is using google
'password' => bcrypt('my-google'), // fill password by whatever pattern you choose
]);
Auth::login($newUser);
return redirect('/dashboard');
}
}
catch (Exception $e)
{
dd($e->getMessage());
}
}
}
Step 2: Create the routes
Add the routes below:
use App\Http\Controllers\Auth\GoogleSocialiteController;
Route::get('auth/google', [GoogleSocialiteController::class, 'redirectToGoogle']); // redirect to google login
Route::get('callback/google', [GoogleSocialiteController::class, 'handleCallback']); // callback route after google account chosen
Step 3: Create the “Login With Google” button
Create the button in the login view. The view can be found in resources/views/auth/login.blade.php
.
// resources/views/auth/login.blade.php
<a href="{{ url('auth/google') }}" class="bg-blue-600 text-white rounded-md px-4 py-2 ml-2">
Google Login
</a>
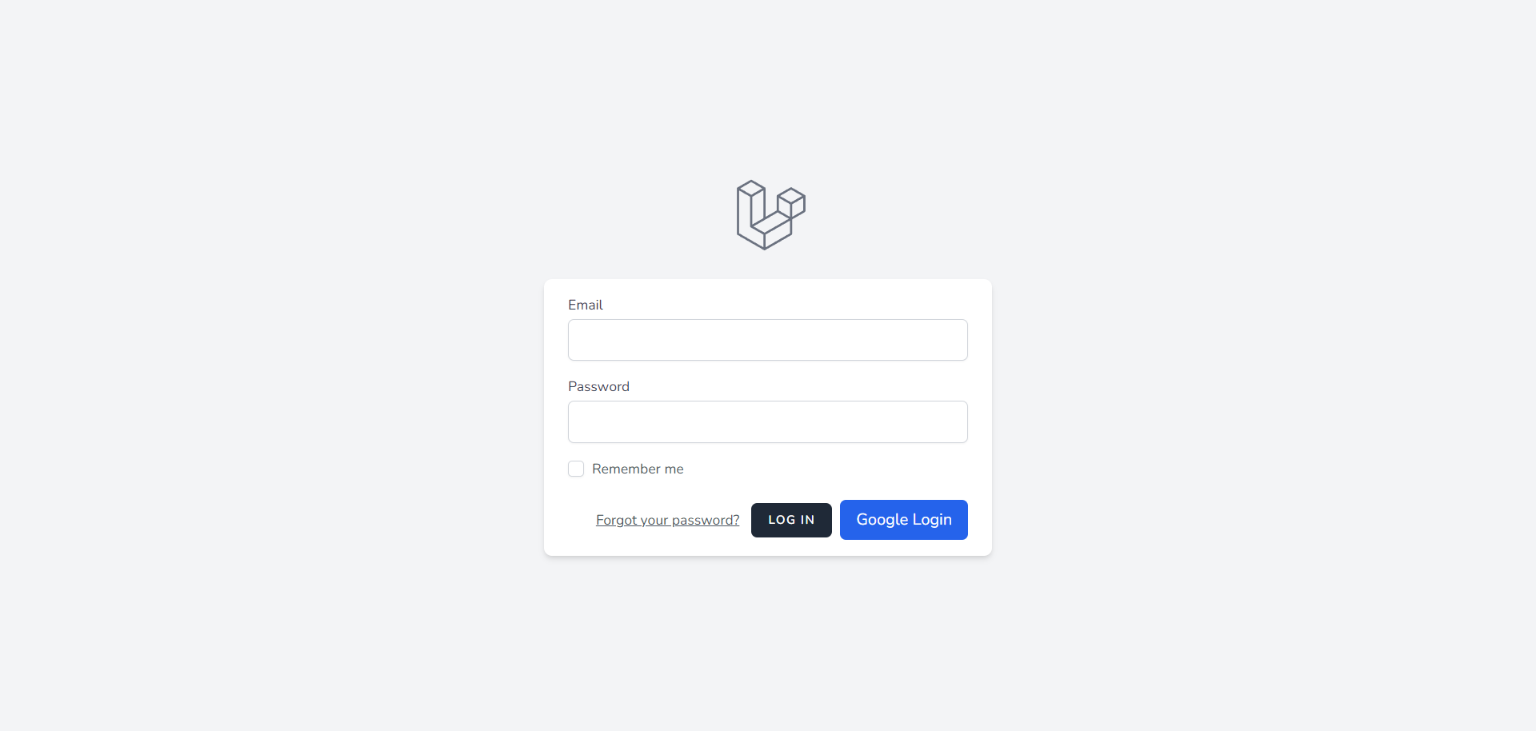
Test It
When the button clicked it will redirect to Google account options
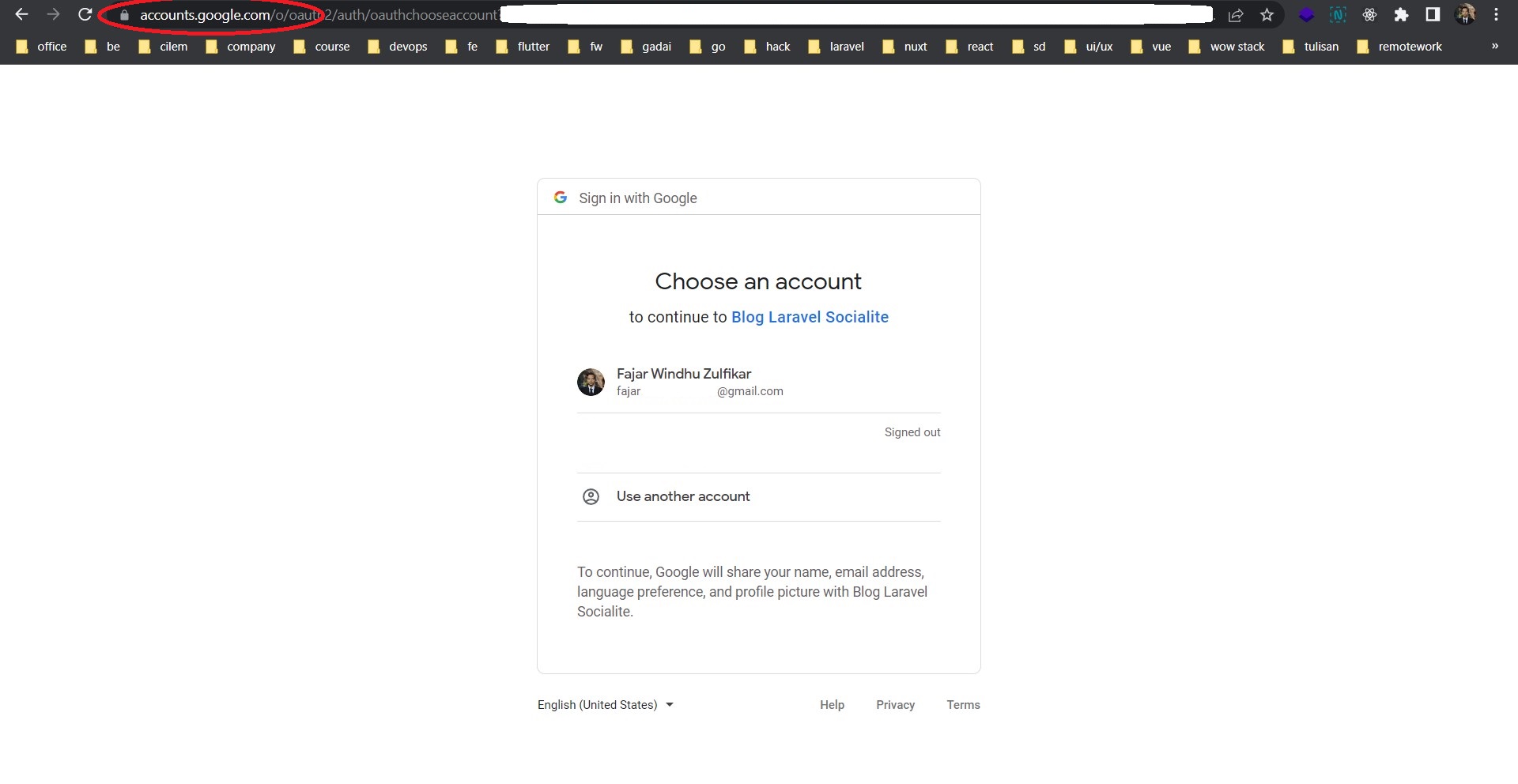
After we choose an account, it will redirect to our web /dashboard
.
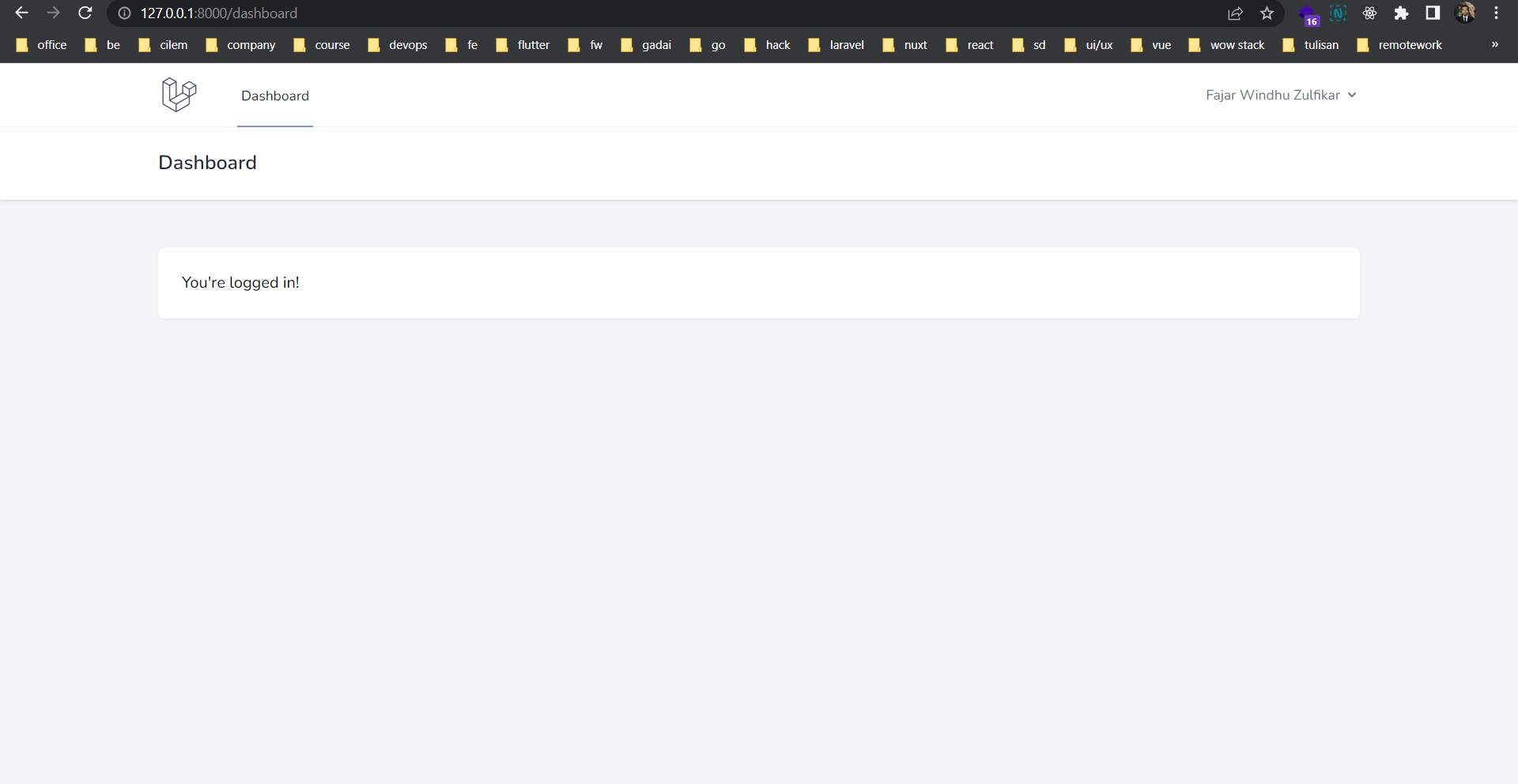
When we check at our users
table, we found our email registered and the social_id
filled

Conclusions
That’s it. We have successfully build an authentication feature with login with Google option. Here we use laravel/socialite package, integrate it with our database, create a controller, create the endpoints, create the button, and done.
💻 A repo for this example case can be found here fajarwz/blog-google-oauth-laravel-socialite.