Clean coding is an important aspect of software development that aims to create code that is readable, maintainable, and efficient. The goal of clean coding is to make the code as easy to understand as possible, both for the developers who wrote it and for those who will be working with it in the future. In this article, we will discuss some key concepts of clean coding, including meaningful naming, code commenting, and code organization.
Meaningful Naming
One of the most important aspects of clean coding is the use of meaningful naming. This refers to the practice of using clear and descriptive names for variables, functions, and classes. When names are meaningful, it is easier to understand what the code is doing, and to make changes to it when necessary.
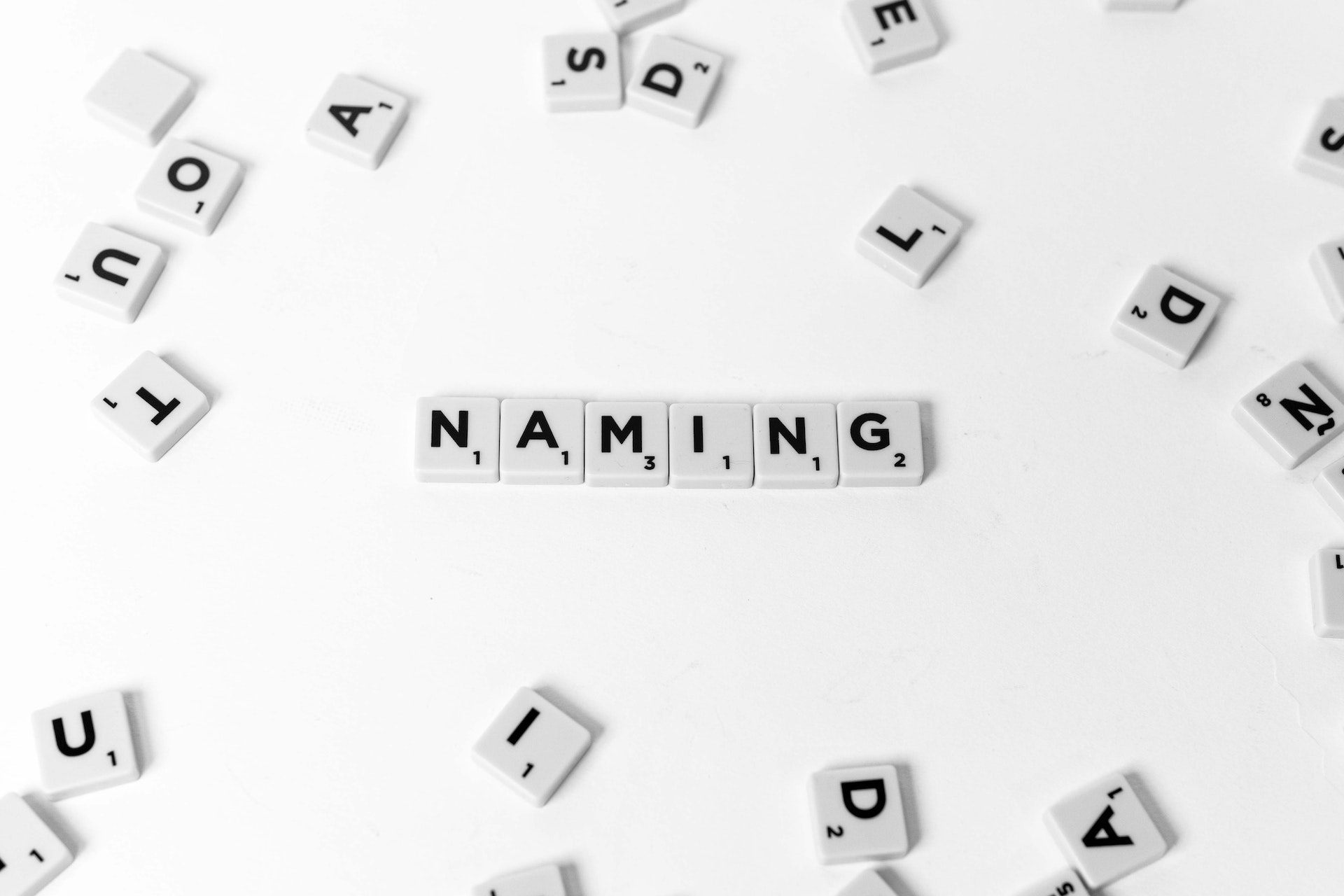
For example, consider the following code snippet:
$x = 5;
$y = 10;
$z = $x + $y;
This code snippet is simple, but the variable names are not meaningful. It is unclear what the purpose of x, y, and z is. Now consider the following code snippet:
$firstNumber = 5;
$secondNumber = 10;
$sum = $firstNumber + $secondNumber;
This code snippet is much more readable and easier to understand. The variable names are meaningful, and it is clear what the code is doing.
Here are some of the reasons why meaningful naming is so important:
Improves readability
Meaningful naming makes the code more readable and easier to understand, even for someone who is not familiar with the code. It helps to avoid confusion and ambiguity, which can lead to errors and bugs in the code.
Enhances maintainability
Clean code should be maintainable, which means it should be easy to update or modify. Meaningful naming makes it easier to understand the function of the code, making it simpler to modify or refactor in the future.
Facilitates collaboration
Meaningful naming helps team members understand what the code is doing and reduces the amount of communication needed to explain the code’s purpose. This can be especially helpful when working on larger projects with multiple developers.
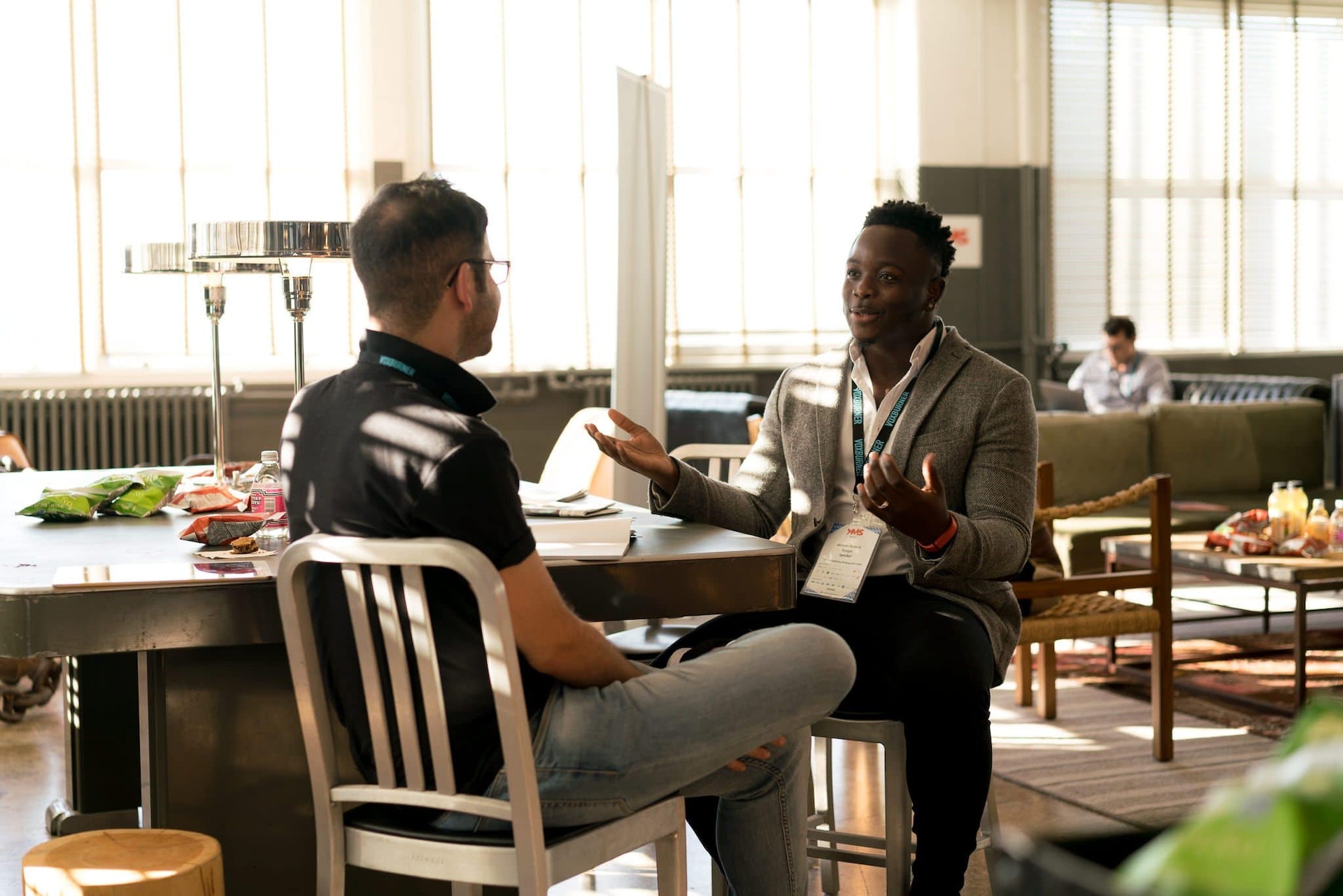
Promotes consistency
By using consistent naming conventions throughout the codebase, developers can improve the code’s overall organization and clarity. This can make it easier for developers to navigate the code, reducing the amount of time it takes to understand how different parts of the code relate to each other.
Improves error detection
When variable names, function names, and class names are meaningful, it is easier to spot errors or issues in the code. This can make it simpler to identify bugs and resolve them more quickly, leading to more efficient debugging and testing processes.
Meaningful Naming vs. Code Commenting
While meaningful naming is important, it is not always sufficient on its own. In some cases, it may be necessary to include comments in the code to explain what is happening. However, code commenting should not be used as a replacement for meaningful naming. If the code is well-written and the variable names are meaningful, comments should be unnecessary.
Here’s an example code snippet that demonstrates the importance of meaningful naming and how it can reduce the need for excessive code commenting:
// Example 1: Poor Naming, Excessive Commenting
// Function to calculate the square of a number
function sq(n) {
// Returns the square of the input number
return n * n;
}
// Main program
const x = 5;
// Calculate the square of the input number and store it in the variable y
const y = sq(x);
// Print the result
console.log(`The square of ${x} is ${y}.`);
// Example 2: Meaningful Naming, Minimal Commenting
// Function to calculate the square of a number
function calculateSquare(number) {
return number * number;
}
// Main program
const inputNumber = 5;
const squareOfInputNumber = calculateSquare(inputNumber);
console.log(`The square of ${inputNumber} is ${squareOfInputNumber}.`);
In the first example, the function name sq
is not very descriptive, and the comments have to explain what the function does and how to use it. In the main program, the variable names x and y are also not very clear, requiring additional comments to explain their purpose.
In the second example, the function name calculateSquare is much more descriptive, making it clear what the function does without any additional comments. In the main program, the variable names inputNumber and squareOfInputNumber are also very clear and don’t require any additional comments to explain their purpose.
Overall, by using meaningful naming conventions, developers can minimize the need for additional comments, making the code simpler, more organized, and easier to understand.
While both practices are essential, meaningful naming is generally preferred over code commenting. Here are some reasons why:
Clarity
Meaningful naming provides clarity and makes the code easier to read and understand. Good naming conventions can eliminate the need for additional comments that only restate what the code is doing. With descriptive naming, developers can easily understand the code’s purpose and how it works without the need for extra comments.
Simplicity
Code that is self-explanatory is often more straightforward than code that requires additional comments. Comments can add unnecessary complexity to the code and create additional work for developers.
Maintenance
Meaningful naming makes code more maintainable by making it easier to modify or update the code. If a variable, function, or class has a clear name that reflects its purpose, developers can quickly understand how it fits into the codebase and how it interacts with other components.
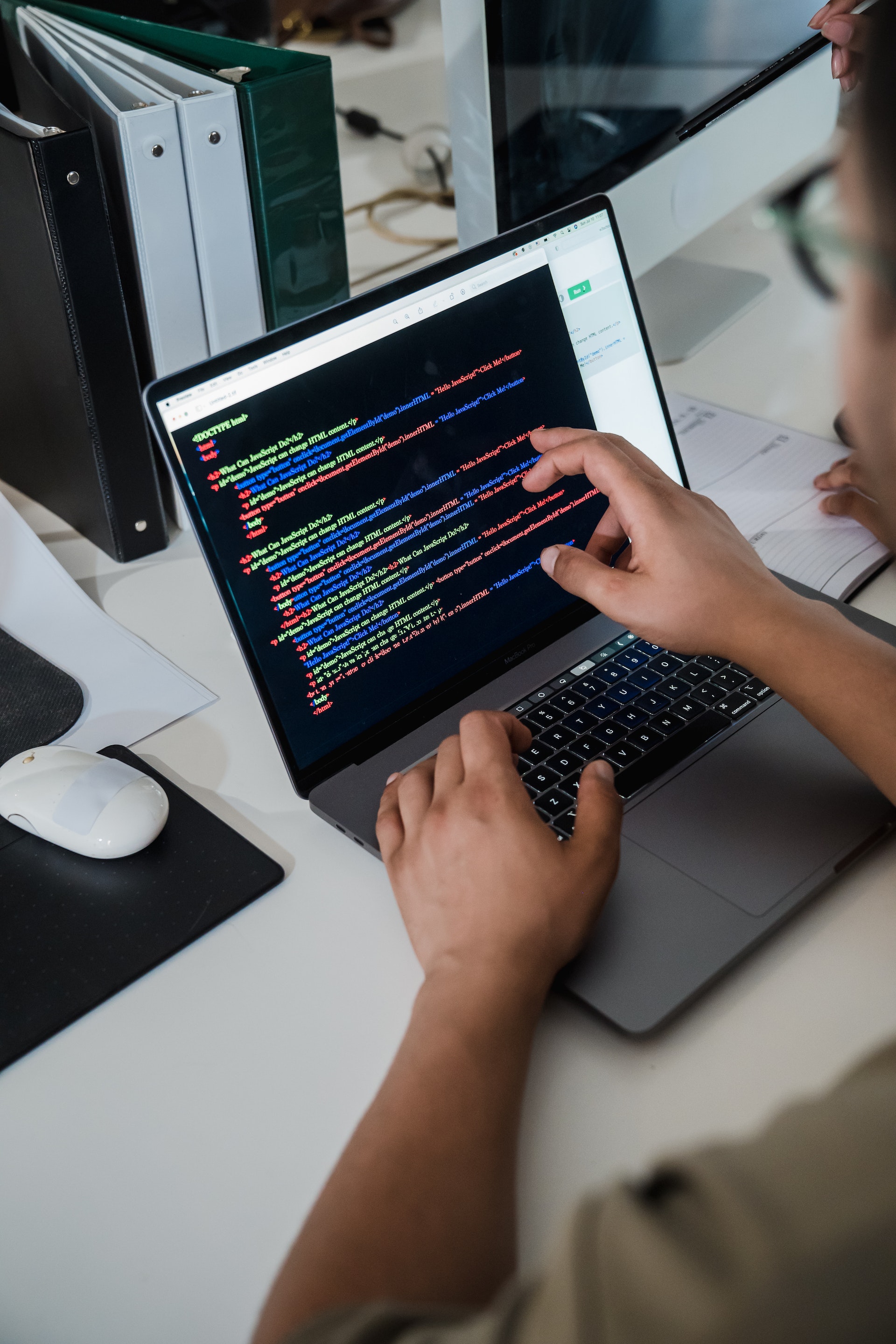
Consistency
Consistent naming conventions make code more organized and easier to navigate. When developers use the same naming conventions throughout the codebase, it is easier to locate functions, variables, and classes that serve a specific purpose.
However, there are times when code commenting is necessary. For instance, if a section of the code is particularly complex, adding comments can help other developers understand its purpose. Comments can also be useful in explaining a particularly tricky or unconventional piece of code.
Code Organization
Another important aspect of clean coding is code organization. This refers to the practice of organizing code in a way that is easy to navigate and understand. Well-organized code should be divided into meaningful sections, with related functions and classes grouped together.
The Use of Modules or Packages
One common approach to code organization is the use of modules or packages. Modules are self-contained sections of code that perform a specific task. By dividing the code into modules, it is easier to understand what each section of code is doing, and to make changes to the code without affecting other parts of the system.
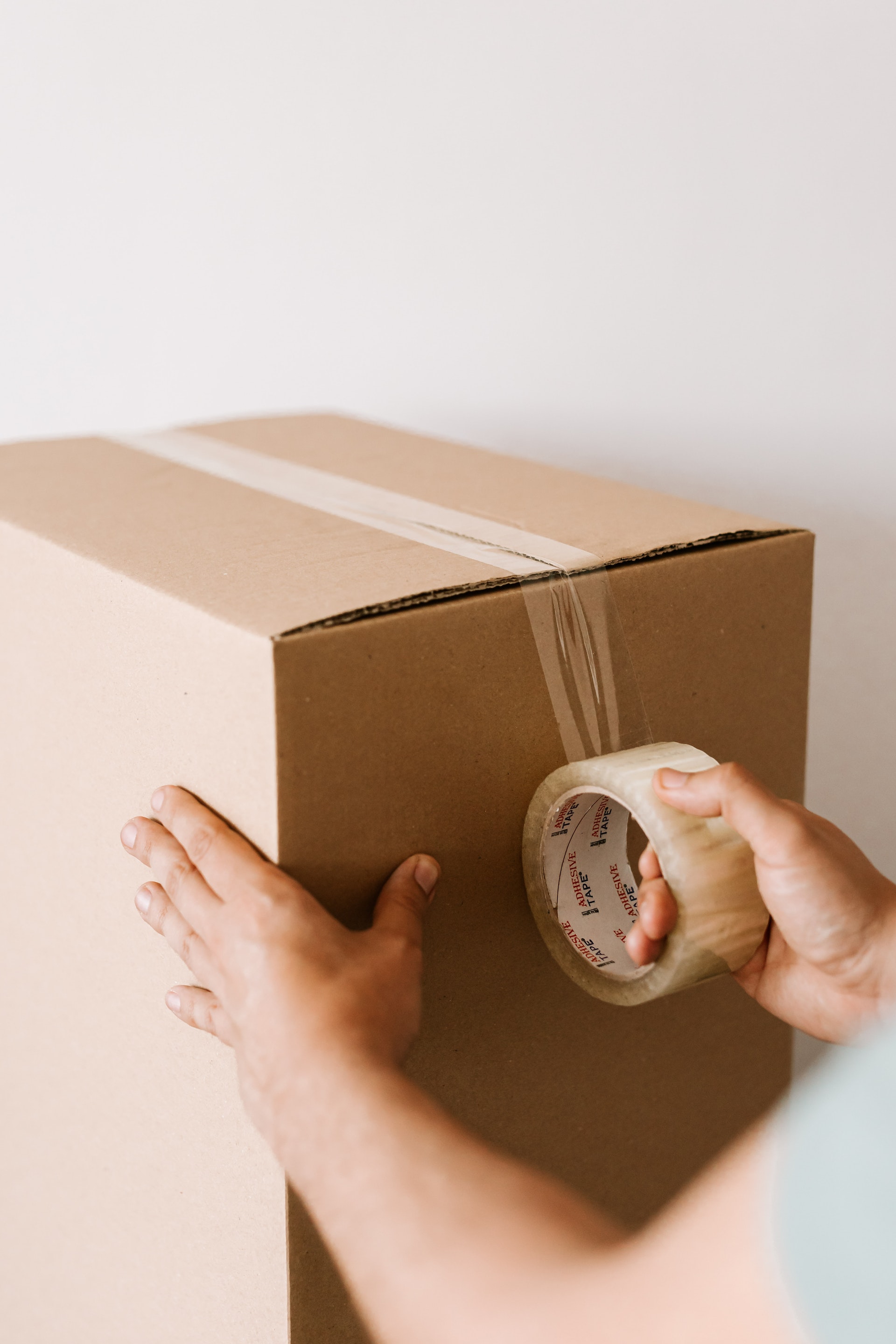
Design Patterns
Another approach to code organization is the use of design patterns. Design patterns are reusable solutions to common software development problems. By using well-known design patterns, it is possible to create code that is more maintainable and easier to understand.
Conclusion
Clean coding is an important aspect of software development that aims to create code that is readable, maintainable, and efficient. By using meaningful naming, organizing code effectively, and minimizing code commenting, it is possible to create code that is easier to understand and maintain over time. While clean coding requires some extra effort on the part of the developer, it is well worth the investment in the long run.